"ReactJS Mastery: A Collection of Interview
Questions and Expert Answers"
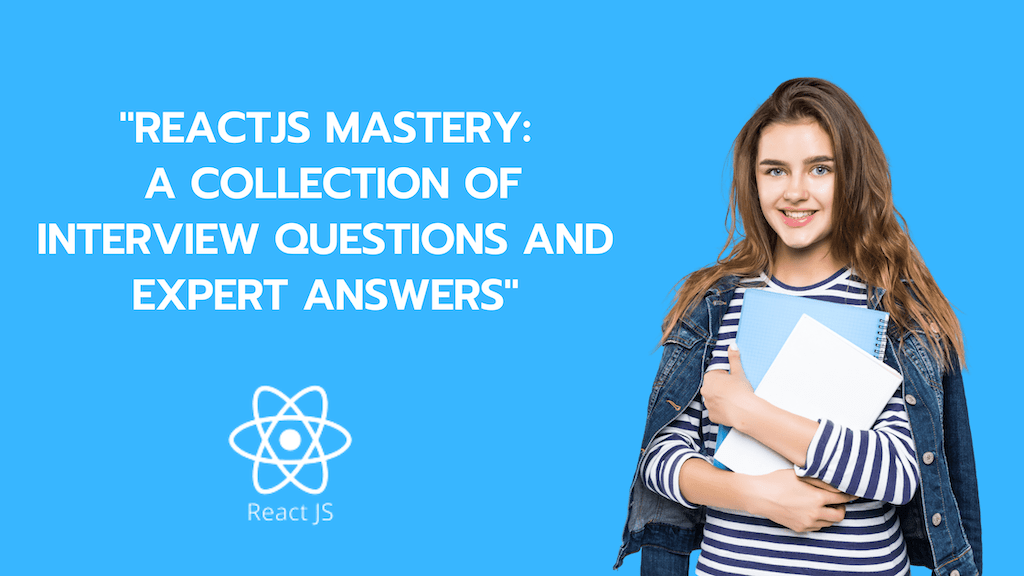
1. What is ReactJS and how does it differ from
other JavaScript libraries and frameworks?
React is a JavaScript library for
building user interfaces. It allows developers to build reusable UI components
and manage the state of their applications in an efficient way. It differs from
other libraries and frameworks, such as Angular or Vue, in that it focuses
solely on the view layer of an application, allowing developers to easily
integrate it with other technologies for the rest of their stack.
2. Can you explain the concept of virtual DOM in
React?
The virtual DOM is a concept unique
to React. When a component's state changes, React will first update the virtual
DOM, which is a lightweight in-memory representation of the actual DOM. It then
compares the virtual DOM with the actual DOM and makes the minimum number of
changes necessary to bring the actual DOM in line with the virtual DOM. This
improves performance by reducing the number of expensive DOM operations that
need to be performed.
3. Can you explain the component lifecycle methods
in React?
React components have several
lifecycle methods that are called at specific points in the component's
lifecycle. Some of the most commonly used methods include:
·
- componentDidMount: called after the
component has been rendered to the DOM
·
- componentDidUpdate: called after the
component's state or props have been updated
·
- the component will unmount: called before
the component is removed from the DOM
·
-
shouldComponentUpdate: called before
the component is re-rendered and allows developers to prevent unnecessary
re-renders.
4. How do you handle events in React?
In React, events are handled using
event handlers. An event handler is a function that is passed as a prop to a
component and is called when a specific event occurs within that component.
For example, to handle a button click in React, you would pass an onClick prop
to the button component, and assign it a function that will be called when the
button is clicked.
5. How do you pass data from a parent component to
a child component in React?
Data is passed from a parent
component to a child component in React using props. Props are a way for a
parent component to pass data to its child component. The child component can
then access this data through the component's props object. For example, a
parent component can pass a name prop to a child component, which the child
component can then use to display the name.
6. What is the significance of the state in React?
The state in React represents the
data or variables that determine a component's behavior or render information
to the user. It allows a component to keep track of information that may change
over time, such as user input or data received from an API. The component can
update its own state using the setState() method and re-render itself to
reflect the changes.
7. How do you use React Router to handle
client-side routing?
React Router is a library that allows
developers to handle client-side routing in their React applications. It
provides a way to declaratively map different URL paths to different
components, allowing for a single-page application experience. Developers can
use the component to define specific paths and the component that
should be rendered for that path. They can also use the component
to create links that will navigate to different routes within the application.
8. How do you manage the state of a form in React?
Managing the state of a form in React
can be done by creating a component that holds the state of the form fields and
handles the form's submission. The component can use the onChange event to
update the state of the form fields as the user interacts with them. When the
form is submitted, the component can access the current state of the form
fields and use it for further processing.
9. What are Higher-Order Components (HOC) in React?
A Higher-Order Component (HOC) is a
pattern in React that allows developers to reuse component logic. A HOC is a
function that takes a component as an argument and returns a new component with
additional functionality. HOCs can be used to add props, behavior, or lifecycle
methods to a component without modifying its original implementation. They are
a way to share common functionality across multiple components, and can also be
used for authentication and authorization.
10. What are the benefits of using React with a tool
like Redux?
React is a great library for building
user interfaces, but it can become complex when managing the state of a large
and dynamic application. Redux is a library that complements React by providing
a centralized store for the application's state and a set of strict rules for
updating the state. This makes it easier to manage the state of an application,
especially when dealing with asynchronous data, and also facilitates debugging
and testing.
11. How do you handle server-side rendering in
React?
Server-side rendering (SSR) is the
process of rendering a React application on the server and sending the
fully-rendered HTML to the client. This can improve the initial load time of a
React application, as well as make it more SEO-friendly. To handle SSR in
React, you can use a library such as React-Router or Next.js, which provide the
tools to handle the server-side rendering and client-side hydration of the
application.
12. How do you test a React component?
There are several libraries and tools
available for testing React components, such as Jest and Enzyme. Jest is a
testing framework that provides a way to run unit tests, while Enzyme is a
testing utility that makes it easier to interact with and assert the output of
React components. Together, these tools allow developers to write unit tests
for their components and ensure that they are functioning as expected.
13. How do you handle errors in a React
application?
Errors in a React application can be handled by using a global
error boundary. A global error boundary is a component that can catch errors
that occur anywhere in the application. It can be used to display a fallback UI
when an error occurs and log the error for debugging purposes.
14. What is the difference between state
and props in React?
State and props are both used to store and manage data in a
React component, but they serve different purposes. Props are used to pass data
from a parent component to a child component, and are immutable, meaning that
the child component cannot modify them. State, on the other hand, is used to
store data that is specific to a component and can be modified by the component
itself.
15. How do you use the Context API in
React?
The Context API is a way to share data between components in a React
application, without having to pass props down through multiple levels of
components. It allows developers to create a context object and provide it to a
component, which can then access the data stored in the context object. It's
useful in situations where the data needs to be shared across many components
and passed down through many levels.
16. What is the use of a key in React?
A
key is a special attribute that can be added to elements in a React component's
JSX code. It is used to identify each element in a list of elements so that
React can keep track of which elements have been added, removed, or modified.
This is important when working with lists, as it allows React to efficiently
update the list without re-rendering the entire list.
17. How do you use the use effect hook in
React?
The use effect hook is a way to run side effects in a functional
component in React. It allows you to synchronize a component with an external
system, and to perform a cleanup when the component unmounts. use effect takes a
function as an argument, and this function will be called after every render,
with an optional cleanup function that will be called before the component
unmounts.
18. How do you use the seducer hook in
React?
The seducer hook is a way to manage the state of a component in a
functional component in React. It allows you to manage the state of a component
in a similar way to how the Redux library manages the state of an application.
useReducer takes two arguments, the first being a reducer function that defines
how the state should be updated, and the second being the initial state.
19. How do you optimize the performance
of a React application?
There are several ways to optimize the performance of a
React application, such as:
·
- Using the shouldComponentUpdate
lifecycle method to prevent unnecessary re-renders
·
- Using the user memo hook to avoid
recomputing expensive calculations
·
- Using the user callback hook to avoid
unnecessary re-creation of callback functions
·
- Using the React DevTools to identify
and troubleshoot performance issues
·
- Using a virtualized list library for
handling large lists of data
20. How do you use the use context hook in
React?
The user context hook is a way to access the context data in a functional
component in React. It allows you to access the context data without having to
pass the context data down through multiple levels of components. use context takes the context object as an argument and returns the current context value.
You can use this value to render the context data and update the context data.